Every library part described with GDL has scripts,
which are lists of the actual GDL commands that construct the 3D shape and the 2D symbol.
Library parts also have a description for quantity calculations.
Master script commands will be executed before each script.
The 2D script contains parametric 2D drawing description.
The binary 2D data of the library part (content of the 2D symbol window) can be referenced
using the FRAGMENT2 command.
If the 2D script is empty, the binary 2D data will be used to display the library part on the floor plan.
The 3D script contains a parametric
3D model description. The binary 3D data
(which is generated during an import or export operation) can be referenced using the BINARY command.
The Properties script contains
components and descriptors used in element, component and zone lists. The binary properties data
described in the components and descriptors section of the library
part can be
referenced using the BINARYPROP command.
If the properties script and the master script are empty, the binary properties data will be used during the list process.
The User Interface script allows the user
to define input pages that can be used to edit the parameter values in place of the normal parameter list.
In the Parameter script, sets of possible values can be defined for the library part parameters.
The parameter set in the Parameters section are used as defaults in the library part settings
when placing the library part on the plan.
In the Forward Migration script you can define the conversion logic which can convert placed instances of older
elements.
In the Backward Migration script you can define a backward conversion to an older version of an element.
The Preview picture is displayed in the library part settings dialog box when browsing the active library.
It can be referenced by the PICTURE and PICTURE2 commands from the 3D and 2D script.
provides a helpful environment to write GDL scripts, with on-the-fly visualization, syntax and error checking.
Analyze, Deconstruct and Simplify
No matter how complex, most objects you wish to create can be broken down into building blocks of simple geometric shapes.
Always start with a simple analysis of the desired object and define all the geometric units that compose it.
These building blocks can then be translated into the vocabulary of the GDL scripting language.
If your analysis was accurate, the combination of these entities will form the desired object.
To make the analysis, you need to have a good perception of space and at least a basic knowledge of descriptive geometry.
![]() |
Window representations with different levels of sophistication |
To avoid getting discouraged early on in the learning process, start with objects of defined dimensions
and take them to their simplest but still recognizable form.
As you become familiar with basic modeling, you can increase the level of sophistication and get closer to the ideal form.
Ideal does not necessarily mean complicated.
Depending on the nature of the architectural project, the ideal library part could vary from basic to refined.
The window on the left in the above illustration fits the style of a design visualization perfectly.
The window on the right gives a touch of realism and detail which can be used later in the construction documents phase of the project.
Elaboration
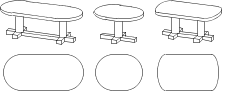
Depending on your purpose, your custom parametric objects may vary in elaboration.
Custom objects for internal studio use may be less refined than the ones for general use or for commercial distribution.
If your symbols have little significance on the floor plan, or if parametric changes do not need to appear in 2D,
then you can omit parametric 2D scripts.
Even if parametric changes are intended to be present in 2D, it is not absolutely necessary to write a parametric 2D script.
You can perform parametric modifications in the 3D Script window or use the 3D top view of the modified object as a new symbol
and save the modified object under a new name.
Parametric changes to the default values will result in several similar objects derived from the original.
The most complex and sophisticated library parts consist of parametric 3D descriptions with corresponding parametric 2D scripts.
Any changes in the settings will affect not only the 3D image of the object, but also its floor plan appearance.
Entry Level
These commands are easy to understand and use.
They require no programming knowledge, yet you can create very effective new objects using only these commands.
Simple Shapes
Shapes are basic geometric units that add up to a complex library part.
They are the construction blocks of GDL. You place a shape in the 3D space by writing a command in the GDL script.
A shape command consists of a keyword that defines the shape type and some numeric values or alphabetic parameters that define its dimensions.
The number of values varies by shape.
In the beginning, you can omit using parameters and work with fixed values only.
You can start with the following shape commands:
In 3D:
In 2D:
LINE2
,
RECT2
,
POLY2
,
CIRCLE2
,
ARC2
Coordinate Transformations
Coordinate transformations are like moving your hand to a certain place before placing a construction block.
They prepare the position, orientation and scale of the next shape.
BLOCK 1, 0.5, 0.5 ADDX 1.5 ROTY 30 BLOCK 1, 0.5, 0.5
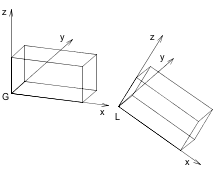
The 3D window of the library part will optionally show you the home (G = global) and the current (L = local) position of the coordinate
system for any object present.
The simplest coordinate transformations are as follows:
In 3D:
ADDX
,
ADDY
,
ADDZ
,
ROTX
,
ROTY
,
ROTZ
In 2D:
The commands starting with ADD will move the next shape, while the ROT commands will turn it around any of its axes.
Intermediate Level
These commands are a bit more complex, not because they expect you to know programming,
but simply because they describe more complex shapes or more abstract transformations.
In 3D:
PRISM_
,
CPRISM_
,
SLAB
,
SLAB_
,
CSLAB_
,
TEXT
In 2D:
HOTSPOT2
,
POLY2_
,
TEXT2
,
FRAGMENT2
These commands usually require more values to be defined than the simple ones.
Some of them require status values to control the visibility of edges and surfaces.
Coordinate Transformations
In 3D:
On top of the entry level transformations
MULX
,
MULY
,
MULZ
,
ADD
,
MUL
,
ROT
In 2D:
On top of the entry level transformations
Example code:
PRISM 4, 1, 3, 0, 3, 3, -3, 3, -3, 0 ADDZ -1 MUL 0.666667, 0.666667, 1 PRISM 4, 1, 3, 0, 3, 3, -3, 3, -3, 0 ADDZ -1 MUL 0.666667, 0.666667, 1 PRISM 4, 1, 3, 0, 3, 3, -3, 3, -3, 0 |
… and it’s 3D view:
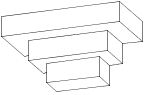
The transformations starting with MUL will rescale the subsequent shapes by distorting circles into ellipses or spheres into ellipsoids.
If used with negative values, they can be used for mirroring. The commands in the second row affect all three dimensions of space at the same time.
Advanced Level
These commands add a new level of complexity either because of their geometric shape, or because they represent GDL as a programming language.
In 3D:
BPRISM_ |
BWALL_ |
CWALL_ |
XWALL_ |
CROOF_ |
FPRISM_ |
SPRISM_ |
|
EXTRUDE |
PYRAMID |
REVOLVE |
RULED |
SWEEP |
TUBE |
TUBEA |
COONS |
MESH |
MASS |
||
LIGHT |
PICTURE |
There are shape commands in this group which let you trace a spatial polygon with a base polygon to make smooth curved surfaces.
Some shapes require material references in their parameter list.
By using cutting planes, polygons and shapes, you can generate complex arbitrary shapes out of simple shapes.
The corresponding commands are CUTPLANE
,
CUTPOLY
,
CUTPOLYA
,
CUTSHAPE
and
CUTEND.
In 2D:
PICTURE2
,
POLY2_A
,
SPLINE2
,
SPLINE2A
Flow Control and Conditional Statements
These commands should be familiar to anyone who has ever programmed a computer,
but they are basic enough that you can understand them without prior programming experience.
They let you make repetitive script parts to place several shapes with little scripting, or let you make decisions based on prior calculations.
Example code:
FOR i = 1 TO 5 PRISM_ 8, 0.05, -0.5, 0, 15, -0.5, -0.15, 15, 0.5, -0.15, 15, 0.5, 0, 15, 0.45, 0, 15, 0.45, -0.1, 15, -0.45, -0.1, 15, -0.45, 0, 15 ADDZ 0.2 NEXT i |
… and it’s 3D view:
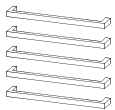
Parameters
At this stage of your expertise, you can replace fixed numeric values with variable names. This makes the object more flexible.
These variables are accessible from the library part’s Settings dialog box while working on the project.
Macro Calls
You are not limited to the standard GDL shapes. Any existing library part may become a GDL shape in its entirety.
To place it, you simply call (refer to) its name and transfer the required parameters to it, just as with standard shape commands.
Expert Level
By the time you have a good understanding of the features and commands outlined above,
you will be able to pick up the few remaining commands that you may need from time to time.
Note
The memory capacity of your computer may limit the file length of your GDL scripts,
the depth of macro calls and the number of transformations.
You will find additional information on the above GDL commands throughout the manual.
HTML format help files are also available with your software, giving a quick overview of the available commands and their parameter structure.